In this post, we delve into the complexities of Textual's widget lifecycle and reactive system, two foundational constructs crucial for building sophisticated, dynamic Textual UI applications. The objective is to elucidate how these components integrate while highlighting the subtle challenges developers frequently encounter when crafting interactive interfaces. We'll enhance this exploration with practical code examples, emphasizing the intersections between lifecycle management and reactivity.
This post is derived from our experiences with the UnderdogCowboy code base. If you are leveraging this code base to construct TUIs for granular tasks—where parts of the workflow are consistently delegated to an AI agent you have developed—this guide will be especially instructive as you begin utilizing Textual.
This blog post does not cover the integration of LLM calls and local storage updates. However, once you have a solid understanding of the core lifecycle phases and reactivity concepts discussed here, you can confidently build to incorporate these integrations, keeping the principles covered in mind as you progress.
As the saying goes in Indonesia, 'Satu per Satu'—one step at a time.
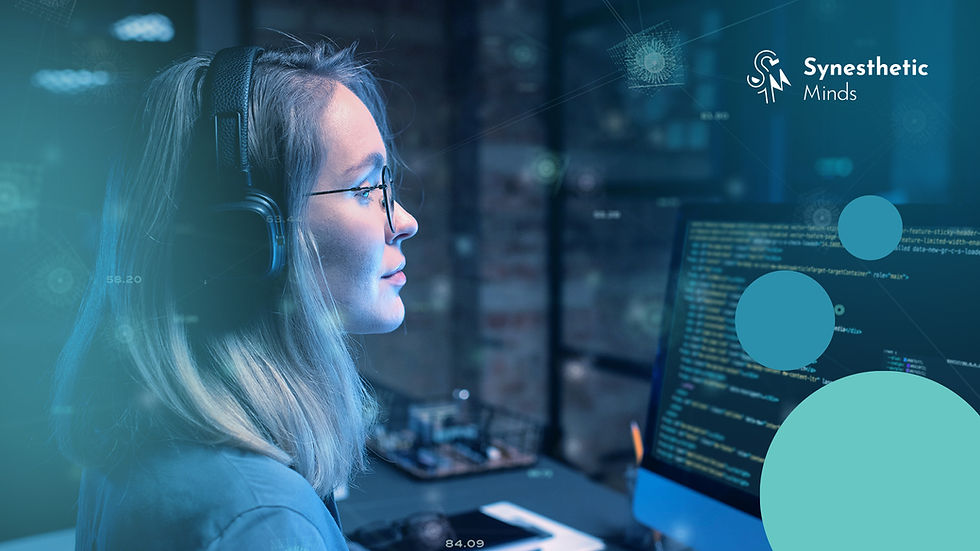
The widget lifecycle: From initialization to full interaction
In Textual, widgets traverse multiple lifecycle phases, each designed to serve a distinct role. A thorough understanding of these phases is imperative for ensuring predictable UI behavior, particularly in dynamic state environments.
1. The initialization phase
init is the constructor method for every widget. It is where initial state configurations, property declarations, and attribute instantiations take place. However, there are inherent limitations to this phase: widgets created here are not yet integrated into the visual layout tree, meaning they are inaccessible for any interaction or querying.
Example:
class MyWidget(Widget):
def __init__(self, categories):
super().__init__()
self.categories = categories # Storing the reference to data for later use
self._init_widgets()
def _init_widgets(self):
"""Initialize widgets, but note that they are not yet part of the interface."""
self.select = Select(options=[("initial", "Initial Category")], id="category-select")
self.loading_indicator = Static("Loading...", id="loading-indicator")
# Widgets here exist in code but aren’t yet composed into the UI.
At this juncture, widgets like select and loading_indicator are instantiated but not yet rendered in the UI. Consequently, attempts to interact with them directly would result in errors.
2. Building the UI layout
The compose method is responsible for yielding widgets to the Textual layout tree. Widgets yielded here are incorporated into the visual hierarchy, thus becoming an integral part of the UI and available for interaction.
Example:
def compose(self) -> ComposeResult:
"""Compose widget layout."""
yield self.select
yield self.loading_indicator
By employing compose, widgets defined earlier are formally added to the layout. At this stage, they are fully integrated into the UI, making them accessible for interaction.
3. Rendering and updating UI state
The render method is responsible for the actual visual representation of a widget. It plays a key role in determining how the widget appears on the screen at any given time, particularly when the internal state changes. This method can be overridden for custom widgets to provide specific rendering behavior.
Example:
def render(self) -> RenderableType:
"""Render the visual representation of the widget."""
if self.is_loading:
return Static("Loading...")
return self.select
In this example, the render method determines the output depending on the current state of the widget. If is_loading is True, it displays a loading message; otherwise, it displays the select widget. The render phase is essential for ensuring the UI is consistently reflective of the internal state and provides a custom appearance as the state evolves.
4. Final setup after layout
on_mount is called once a widget is part of the UI and fully mounted. This phase is the most suitable for initializing components requiring access to the composed layout, such as querying widgets or configuring their initial states and visibility.
Example:
def on_mount(self) -> None:
"""Initialize after mounting."""
self.select = self.query_one("#category-select", Select)
self.loading_indicator.visible = False # Hide loading indicator initially
self.refresh()
By placing assignments and setup actions within on_mount, you guarantee that widgets like select are already part of the layout hierarchy, enabling safe interaction and modification.
The reactive system: Managing state changes dynamically
Textual's reactive system enables widgets to automatically respond to state changes. Reactive properties facilitate UI updates upon state modification, eliminating the need for manually managing transitions. Let's explore where and how reactive variables fit into the broader lifecycle.
Declaring reactive properties
Reactive properties are specialized fields that, when modified, trigger automatic re-renders or the execution of associated watchers. These properties are declared at the class level, allowing for seamless synchronization between internal state and UI representation.
Example:
class SelectCategoryWidget(Static):
selected_category = Reactive[Optional[str]](None)
is_loading = Reactive[bool](False)
categories = Reactive[List[Dict]](default=[])
show_controls = Reactive[bool](False)
In the code above:
selected_category tracks the currently selected category, enabling the UI to respond to changes.
is_loading manages the visibility of the loading indicator.
categories stores the list of categories; updates to this list automatically reflect in the UI.
show_controls determines the visibility of editing controls.
Timing and assignment in reactive variables
Proper timing for assigning reactive variables is crucial. Assigning reactive properties in inappropriate lifecycle phases can result in unexpected errors, such as the inability to locate widgets or inconsistent UI updates.
For instance, initializing a reactive property in init and assigning a value before the widget is fully composed can lead to scenarios where UI elements that depend on that property aren't properly updated because they do not yet exist.
To prevent these issues, assign reactive properties in on_mount—once the layout is complete. This approach allows the layout to be established before the reactive assignment, ensuring that components relying on these properties are ready for interaction.
Example:
class SelectCategoryWidget(Static):
def __init__(self, categories):
super().__init__()
self._categories_reference = categories # Reference for later assignment
def on_mount(self) -> None:
"""Mount the widget and initialize reactive categories."""
self.categories = self._categories_reference # Assign to trigger reactivity safely
In this example, categories reference is created in the init method and assigned to the reactive categories property after the widget is fully mounted. The reason for this pattern is that reactive properties often need to be updated after the widget is mounted to ensure proper synchronization between widgets. When data needs to be passed reactively between widgets, initializing references in init and assigning them in on_mount prevents premature state changes that might affect dependent widgets, ensuring UI components relying on categories are correctly updated.
This is due to the fact that reactivity in Textual is scoped to individual widgets. When passing data between widgets, you're effectively passing a reference to the data wrapped within the reactive property. This nuanced approach to referencing ensures that the data remains consistent across different components. Understanding this subtle detail can save significant time when orchestrating complex widget interactions within a Textual-based system.
This illustrates the critical role of scope and timing in managing reactive variables effectively. When passing data between widgets, reactive properties must be assigned in such a way that ensures all dependent widgets are fully ready. This is why assigning in on_mount is crucial, while having the reference injected in init allows you to access and control it appropriately later on. This pattern ensures that references are properly wrapped in Reactive 'mode,' maintaining consistency across the entire UI.
Watching reactives: Handling changes automatically
Textual allows developers to define watchers for reactive properties. Watchers are methods that execute automatically whenever the reactive property changes, helping maintain UI consistency without manual intervention.
Example of a watcher:
def watch_categories(self, new_value: List[Dict], old_value: List[Dict]) -> None:
"""Watch changes to categories and update options in select."""
if hasattr(self, 'select') and self.select is not None:
options = [("refresh_all", "Refresh All")] + [(cat['name'], cat['name']) for cat in new_value]
self.select.set_options(options)
self.refresh()
This watcher automatically updates the options in select whenever categories changes. Note that the safeguard (hasattr check) is a potential code smell; ideally, if lifecycle phases are properly managed, such checks should be unnecessary. Ensuring that reactive properties are assigned in the correct phase guarantees that widgets like select are always ready when needed, thereby avoiding premature access errors.
Avoiding common pitfalls in reactivity
Accessing widgets too early: Always ensure widgets are fully mounted before interacting with them. Attempting to query or modify widgets in init can lead to AttributeError since the widget isn’t yet integrated into the visual hierarchy.
Reactive properties without proper initialization: Reactive properties should not be assigned or modified before the widget is fully ready. Assign initial values in on_mount to prevent premature updates.
Missing Watchers: If a reactive property is expected to update a UI element but fails to do so, ensure a corresponding watcher is defined. Without watchers, the reactive system lacks instructions on how to handle property changes.
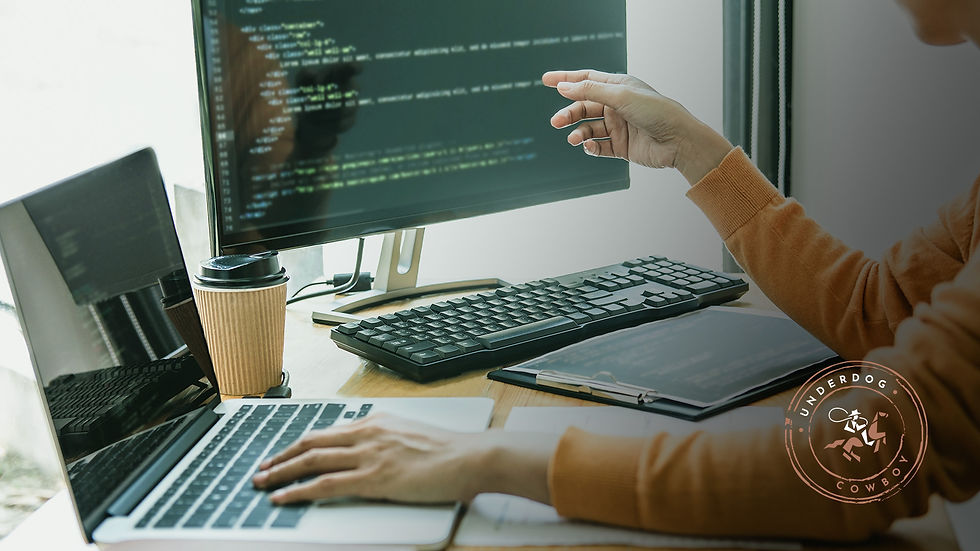
Conclusion
The combination of lifecycle management and the reactive system in Textual is foundational to creating dynamic, responsive UIs. However, small missteps, such as assigning properties prematurely, skipping lifecycle hooks, or mishandling reactive state, can result in challenging bugs.
Key takeaways include:
Utilize init for basic initialization, compose for defining the UI layout, on_mount for finalizing setup after composition, and render for controlling the visual representation of a widget as the state changes.
Assign reactive properties at appropriate lifecycle phases to prevent premature or inconsistent updates.
Employ watchers effectively to maintain a consistent UI state as reactive properties change.
By adhering to these practices, you can fully leverage Textual’s lifecycle and reactive system to develop robust, responsive, and maintainable UIs.
When building Textual Tools around our code base in UnderdogCowboy, it is crucial to maintain a clear mental model of the lifecycle phases, the reactive system, and the appropriate timing for state changes. Ensuring these components fit together seamlessly will help you avoid pitfalls and make your UI more predictable and responsive.
Comments